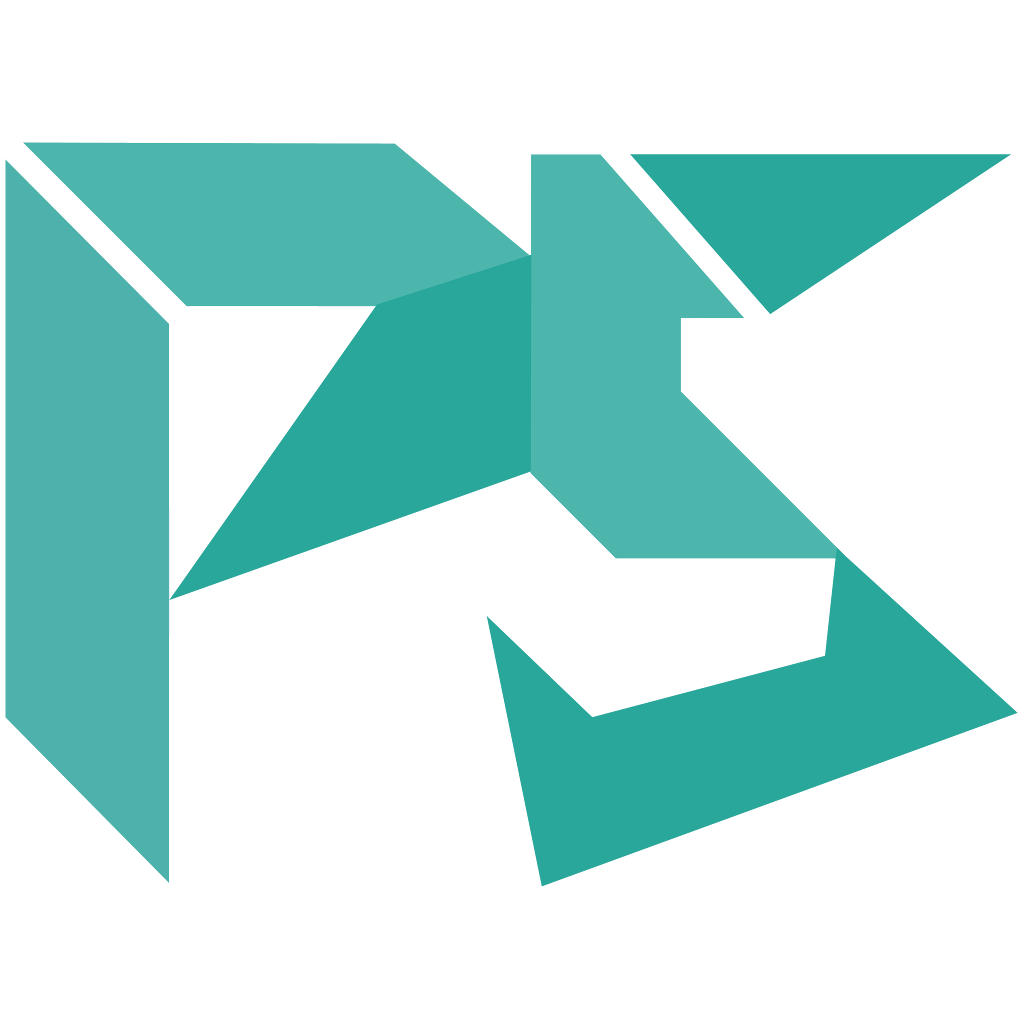
GitHub Actions is a tool for automating tasks in a repository by setting up workflows that run in response to specific triggers. This cheat sheet provides a quick reference for using GitHub Actions, including tips on setting up workflows, writing jobs and tasks, using actions, and working with environment variables. By following these guidelines, you can streamline your development process and save time by automating repetitive tasks.
To set up a workflow, create a new file called main.yml
in the .github/workflows
directory and add the following configuration:
name: Workflow Name
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Task 1
run: <command>
- name: Task 2
run: <command>
This configuration specifies the name of the workflow, the trigger for the workflow (in this case, a push to the main
branch), and the jobs to be run.
A job is a series of tasks that are run in a workflow. To write a job, specify the runs-on
key with the type of machine the job should run on (e.g. ubuntu-latest
or self-hosted
) and the steps
key with the individual tasks to be run:
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Task 1
run: <command>
- name: Task 2
run: <command>
To run a task, use the name
key to give it a descriptive name and the run
key to specify the command to be run:
steps:
- name: Install Dependencies
run: npm install
- name: Run Tests
run: npm test
Actions are pre-built, reusable tasks that can be used in your workflow. To use an action, add a uses
key to your job’s configuration and specify the name of the action:
steps:
- uses: actions/checkout@v2
- name: Install Dependencies
run: npm install
You can also specify specific versions of actions using the @
symbol, e.g. actions/checkout@v2
.
Environment variables are values that can be passed to your workflow at runtime. To set an environment variable, use the env
key in your job’s configuration and specify the name and value of the variable:
jobs:
build:
runs-on: ubuntu-latest
env:
MY_VAR: value
steps:
- name: Task 1
run: <command>
To access an environment variable in a task, use the $
symbol followed by the variable name, e.g. $MY_VAR
.
GitHub Actions is a powerful tool for automating tasks in your repository. By following these tips and using the code examples provided, you can quickly set up and configure your own workflows to streamline your development process.