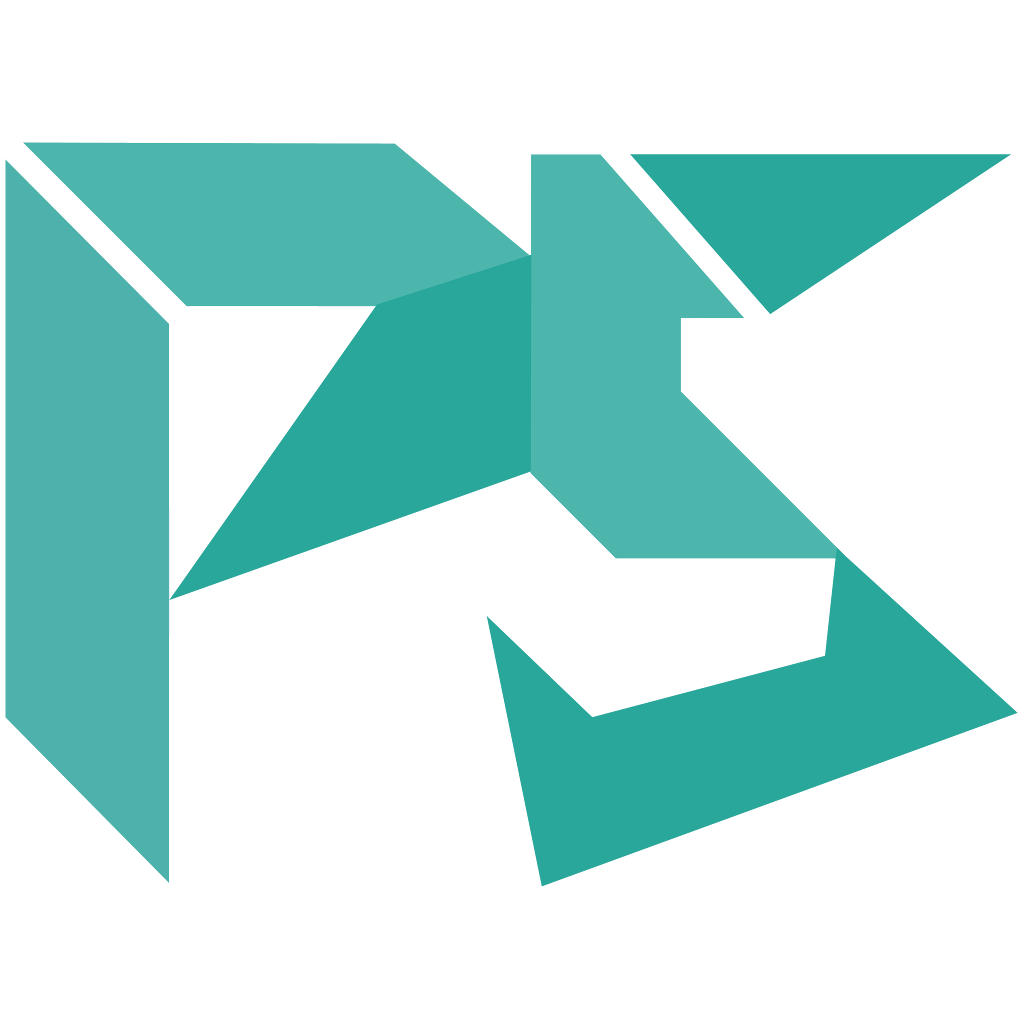
Synthesize lets you make bitmaps without inflating them and in background.
Drawing the exact image shown in the layout can be a difficult task on Canvas. Defining the margins, colors, typeface, text wrapping, etc. is a huge task to accomplish manually. To draw on a canvas you must also define the styles. Generating bitmap from the layouts gives you freedom from the headaches drawing on the canvas.
Here comes Synthesize to the rescue. All you have to do is initialize the layout XML as you would do in any activity or fragment using Synthesize, the only difference is that you can now do it in background.
//Project Level gradle
allprojects {
repositories {
...
maven { url "https://jitpack.io" }
}
}
//Module Level gradle
dependencies{
compile "com.prashantsolanki:Synthesize:0.1.5"
}
Synthesize s= new Synthesize(this,R.layout.resource_id);
//Required
s.setParams(1024,1080);
//Required
s.generateBitmap(new OnBitmapGenerationListener(){
@Override
void onSuccess(Bitmap bitmap){
}
@Override
void onError(Exception e){
}
})
//Optional
s.saveImage();
setLayout(int layoutRes) or setLayout(View v)
: Only required if layoutId has not been set using Constructor. Sets the layout id of the XML to be synthesized.
setParams(int width,int height)
: Sets the dimensions of Output image.
generateBitmap(OnBitmapGenerationListener listener)
: Generates the Bitmap of the given layout.
saveImage() or saveImage(OnSaveListener listener)
: Saves the image in the given path, or the media root if no image path is given.
setOutputQuality(int quality)
: Set the required output quality on the scale of 0 to 100.
setOutputPath(File outputPath)
: Set the path to save the output image.
setCompressionFormat(Bitmap.CompressFormat compressionFormat)
: Supported Compression formats are : JPEG, PNG and WEBP (requires SDK > 19).
setFileName(String fileName)
: Name of the output file.
setLayout(View)
can be used to populate the view before generating the bitmap.
getLayout()
can be used to populate the view after being inflated by Synthesize. It can be helpful when generating bitmap in background thread.